​
Features
​
-
Free and open-source (MIT License);
-
a collection of native C++ classes libraries, to complete std;
-
API close to the .net API with a modern C++ approach and full integration with the std standard;
-
xtd is designed to manage GUI controls and dialogs in pure native mode or with CSS styles.
-
written in efficient, modern C++17 / C++20 with RAII programming idiom
-
and highly portable and available on many different platforms;
​
Getting Started
​
-
Installation provides download and install documentation.
-
Guides provides xtd guides and tutorials.
-
Examples provides some examples.
​
Examples
​
The classic first application 'Hello World'.
​
Console
hello_world_console.cpp:
​
#include <xtd/xtd>
​
using namespace xtd;
​
int main() {
console::background_color(console_color::blue);
console::foreground_color(console_color::white);
console::write_line("Hello, World!");
}
​
CMakeLists.txt:
​
cmake_minimum_required(VERSION 3.3)
project(hello_world_console)
find_package(xtd REQUIRED)
add_sources(hello_world_console.cpp)
target_type(CONSOLE_APPLICATION)
​
Build and run
​
Open "Command Prompt" or "Terminal". Navigate to the folder that contains the project and type the following:
​
xtdc run
​
Output
​
Hello, World!
​
Gui
hello_world_forms.cpp:
​
#include <xtd/xtd>
​
using namespace xtd::forms;
auto main()->int {
auto main_form = form::create("Hello world (message_box)");
auto button1 = button::create(main_form, "&Click me", {10, 10});
button1.click += [] {message_box::show("Hello, World!");};
application::run(main_form);
}
​
CMakeLists.txt:
​
cmake_minimum_required(VERSION 3.3)
project(hello_world_forms)
find_package(xtd REQUIRED)
add_sources(hello_world_forms.cpp)
target_type(GUI_APPLICATION)
​
Build and run
​
Open "Command Prompt" or "Terminal". Navigate to the folder that contains the project and type the following:
​
xtdc run
​
Output
​
Windows:
​
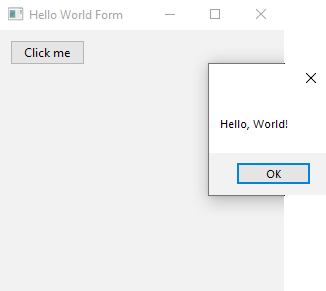
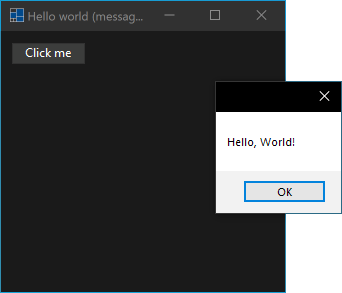
macOS:
​
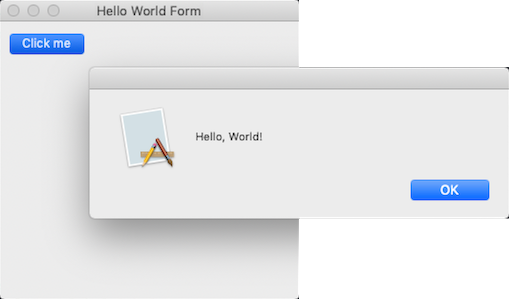

Linux Gnome:
​
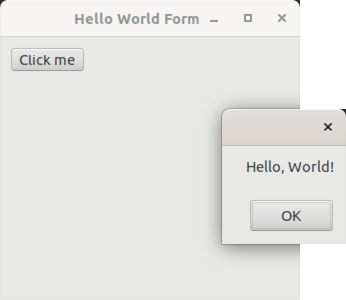

​
Unit test
hello_world_test.cpp:
​
#include <xtd/xtd>
#include <string>
using namespace std;
using namespace xtd::tunit;
namespace unit_tests {
class test_class_(hello_world_test) {
public:
void test_method_(create_string_from_literal) {
string s = "Hello, World!";
valid::are_equal(13, s.size());
assert::are_equal("Hello, World!", s);
}
void test_method_(create_string_from_chars) {
string s = {'H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!'};
valid::are_equal(13, s.size());
string_assert::starts_with("Hello,", s);
string_assert::ends_with(" World!", s);
}
};
}
int main() {
return console_unit_test().run();
}
​
CMakeLists.txt:
​
cmake_minimum_required(VERSION 3.3)
project(hello_world_test)
find_package(xtd REQUIRED)
add_sources(hello_world_test.cpp)
target_type(TEST_APPLICATION)
​
Build and run
​
Open "Command Prompt" or "Terminal". Navigate to the folder that contains the project and type the following:
​
xtdc run
​
Output
​
Start 2 tests from 1 test case Run tests:
SUCCEED hello_world_test.create_string_from_literal (0 ms total)
SUCCEED hello_world_test.create_string_from_chars (0 ms total)
Test results:
SUCCEED 2 tests.
End 2 tests from 1 test case ran. (0 ms total)